If you're wanting to display the numbers, as opposed to plotting them, you have a few options. A very simple one is to use the MSGBOX function for opening a dialog window and displaying a string. You would have to first convert your number(s) to a string representation using functions like INT2STR, NUM2STR, or SPRINTF. Here's an example:
sumsurface = rand; %# Pick a random number
pH = rand; %# Pick another random number
str = {['sumsurface = ' num2str(sumsurface)]; ...
['pH = ' num2str(pH)]}; %# Creates a 2-by-1 cell array of strings
msgbox(str);
and here is the resulting dialog box:
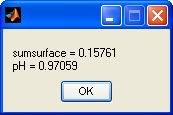
You can also create static text boxes yourself using the UICONTROL function. This would be a better choice if you want to insert the text boxes into an existing GUI. Here's an example of how you could initialize the figure and text boxes for the GUI:
hFigure = figure('Position',[300 300 150 70],...
'MenuBar','none');
hText1 = uicontrol('Style','text','Parent',hFigure,...
'Position',[10 40 130 20],...
'BackgroundColor',[0.7 0.7 0.7]);
hText2 = uicontrol('Style','text','Parent',hFigure,...
'Position',[10 10 130 20],...
'BackgroundColor',[0.7 0.7 0.7]);
Now you can use the handles for the text boxes to update the String
property to whatever you want to display:
set(hText1,'String',['sumsurface = ' num2str(rand)]);
set(hText2,'String',['pH = ' num2str(rand)]);
and here is what the figure looks like:
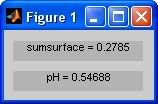