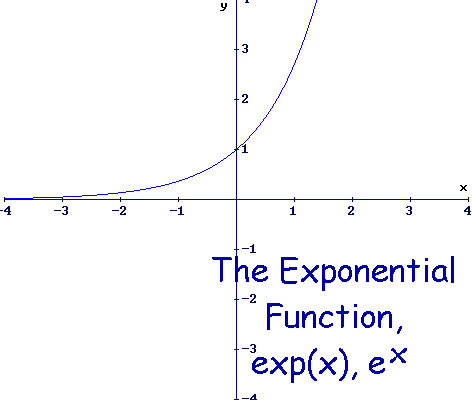
The above graph is from -4 to 4 on the x axis , it's easy to figure out the y value for x=-5 - it's almost 0 - so please consider this when reading the following.
Please see the above graph, it's the graph of the exponential function. See how it grows for x values from -5 to 0? It's not a linear growth but rather it's a bigger and bigger growth while it reaches 0. This is exactly what you need for your animation.
val=Math.exp(intervalMaxValue-intervalLength+((1-(stage.mouseY/stage.stageHeight)) * intervalLength))*scale;
intervalMaxValue-intervalLength is actually 0-5 which would be -5
So we start with -5!
Next
stage.mouseY/stage.stageHeight = 0 when your mouse is at the top of the stage(as stage.MouseY would be 0) and 1 when the mouse is at the Bottom of the stage ( stage.mouseY is then equal to stage.stageHeight so you divide stage.stageHeight by stage.stageHeight which will be 1)
For your effect you want it to be inverted, 1 when you are at the top and 0 when you are at the bottom so that's why you have to use (1-(stage.mouseY/stage.stageHeight))
Now when the mouse is at the top the above formula returns 1 and when it's at the bottom returns 0 ( exactly like you want it to behave , a big spin when it's at the top and a small one when it reaches the bottom )
Let's now take only the Math.exp part and see how it behaves
val=Math.exp(intervalMaxValue-intervalLength+((1-(stage.mouseY/stage.stageHeight)) * intervalLength);
When your mouse is at the TOP the above formula would be -5+((1-(0))*5(<-- 5 is here the intervalLength); this would return 0. Take a look at the graph now, for 0 on the x axis you get 1 on the y axis.
Now let's see what happens when the mouse is at the BOTTOM -5+(1-(1))*5; this would return -5. For -5 on the x axis you get something very close to 0 on the y axis as you can see in the image above.
Let's see what happens when the mouse is at the MIDDLE -5+(1-(0.5))*5; this would return -5+2.5 which would be -2.5.For -2.5 on the x axis you have something more closer to 0 on the y axis rather than on 1.This is what the exponential function does.
As you can see, on this interval [-5,0] the exponential function generates a y value from 0 to 1 and this value's growth is bigger as it approaches the x value of 0.
Considering that it generates a value from 0 to 1, that drops a lot while going to -5, you now multiply this value with 360 so you will get an angle value out of it.
At the TOP, the angle value is 1*360;
MIDDLE: the Math.exp for -2.5 returns a value somewhere near 0.1, multiply this by 360 and you get 36.If you compare it to the 360 for the TOP of the stage you observer that it sharply fell while the mouse reached the middle.
BOTTOM: Math.exp for -5 returns a value very close to 0. Multiply 0 with 360 and you get no angular movement. So when mouse is at the bottom of the stage the rotation value is extremely close to 0.
Hope this is clear, it was written at 6:53 AM, after a night in the club and a few shots of whiskey :)
Cheers.