If you want to try and make something that looks like the image you linked to, here's some code to help you do it (NOTE: you would first have to download the submission arrow.m by Erik Johnson on the MathWorks File Exchange, which I always like to use for generating arrows of any shape and size):
x = 1; %# X coordinate of arrow start
y = 2; %# Y coordinate of arrow start
theta = pi/4; %# Angle of arrow, from x-axis
L = 2; %# Length of arrow
xEnd = x+L*cos(theta); %# X coordinate of arrow end
yEnd = y+L*sin(theta); %# Y coordinate of arrow end
points = linspace(0,theta); %# 100 points from 0 to theta
xCurve = x+(L/2).*cos(points); %# X coordinates of curve
yCurve = y+(L/2).*sin(points); %# Y coordinates of curve
plot(x+[-L L],[y y],'--k'); %# Plot dashed line
hold on; %# Add subsequent plots to the current axes
axis([x+[-L L] y+[-L L]]); %# Set axis limits
axis equal; %# Make tick increments of each axis equal
arrow([x y],[xEnd yEnd]); %# Plot arrow
plot(xCurve,yCurve,'-k'); %# Plot curve
plot(x,y,'o','MarkerEdgeColor','k','MarkerFaceColor','w'); %# Plot point
And here's what it would look like:
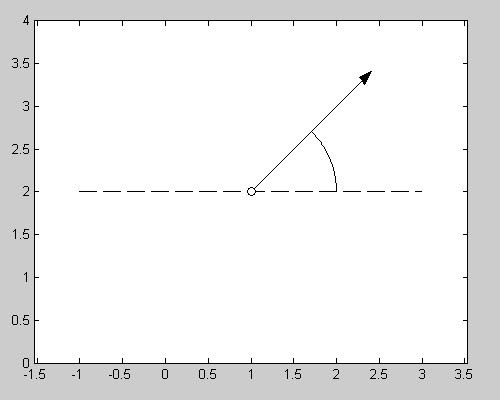
You can then add text to the plot (for the angle and the coordinate values) using the TEXT function.