One possible approach.
In your AndroidManifest.xml add metadata to your Activities to keep the revision or whatever you want to use
<activity android:name="SampleActivity">
<meta-data android:value="$Rev: 164 $" android:name="com.example.version" />
</activity>
then in your Activity
try {
final ActivityInfo ai = getPackageManager().getActivityInfo(getComponentName(), PackageManager.GET_META_DATA);
final String version = (String)ai.metaData.get("com.example.version");
System.out.println("version: " + version);
} catch (NameNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
You may also want to set android:versionName="$Rev$"
in your manifest.
To automate the process of incrementing the version number in android:versionCode
and committing the AndroidManifest.xml
there are various options, building with maven
or ant
could be alternatives but let's do it using Eclipse and ADT.
First, add a builder to your project (My Project -> Properties -> Builders)
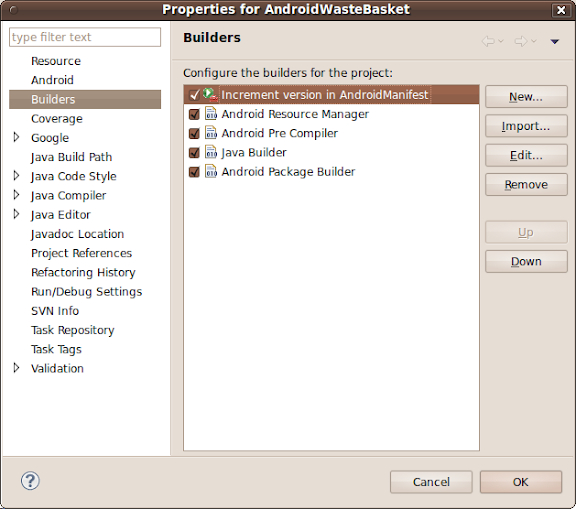
this builder should invoke the android-increment-manifest-version
using the project's AndroidManifest.xml
as the argument
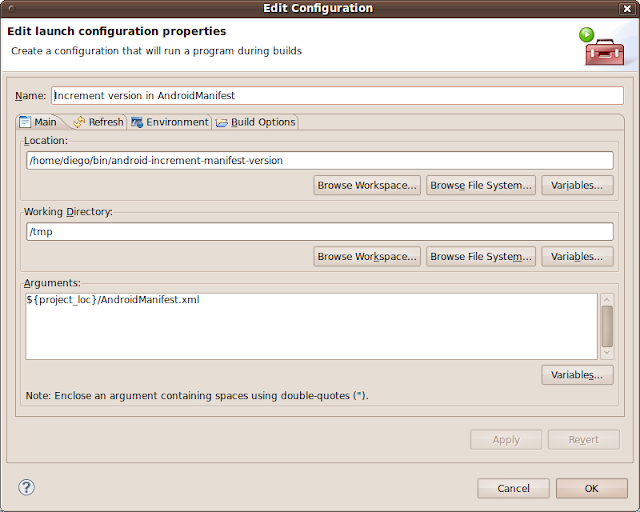
android-increment-manifest-version script is something like
#! /bin/bash
# android-increment-manifest-version:
# increment the version number found in the AndroidManifest.xml file
# (android:versionCode="n") in place and commit it to subversion.
#
# Copyright (C) 2010 Diego Torres Milano - http://dtmilano.blogspot.com
usage() {
echo "usage: $PROGNAME AndroidManifest.xml" >&2
exit 1
}
PROGNAME=$(basename $0)
if [ "$#" -ne 1 ]
then
usage
fi
MANIFEST="$1"
perl -npi -e 's/^(.*android:versionCode=")(\d+)(".*)$/"$1" . ($2+1) . "$3"/e;' $MANIFEST
svn ci -m "Version incremented" $MANIFEST