Here is the SQL end of things, as far as making an output file, thats pretty basic so Ill leave that code up to you. There are other options for executing the query, depends on what you need in your implementation.
edit Can you be a little more specific in what you are looking for? Ill add the code for making a log as well I suppose and write out everything.
edit 2 For an accdb file you will need to use an OleDB connection not a SQL connection as shown in this diagram. I have edited the code below to work with an accdb file. I am not as familiar with this so I can't promise it will work 100% but the only issues should be syntax. By the way none of the inputs are sanitized and it may be better to parametrize the inputs, but since it sounds like you are running this yourself it shouldnt be necessary. If this answers your questions please mark as accepted :)
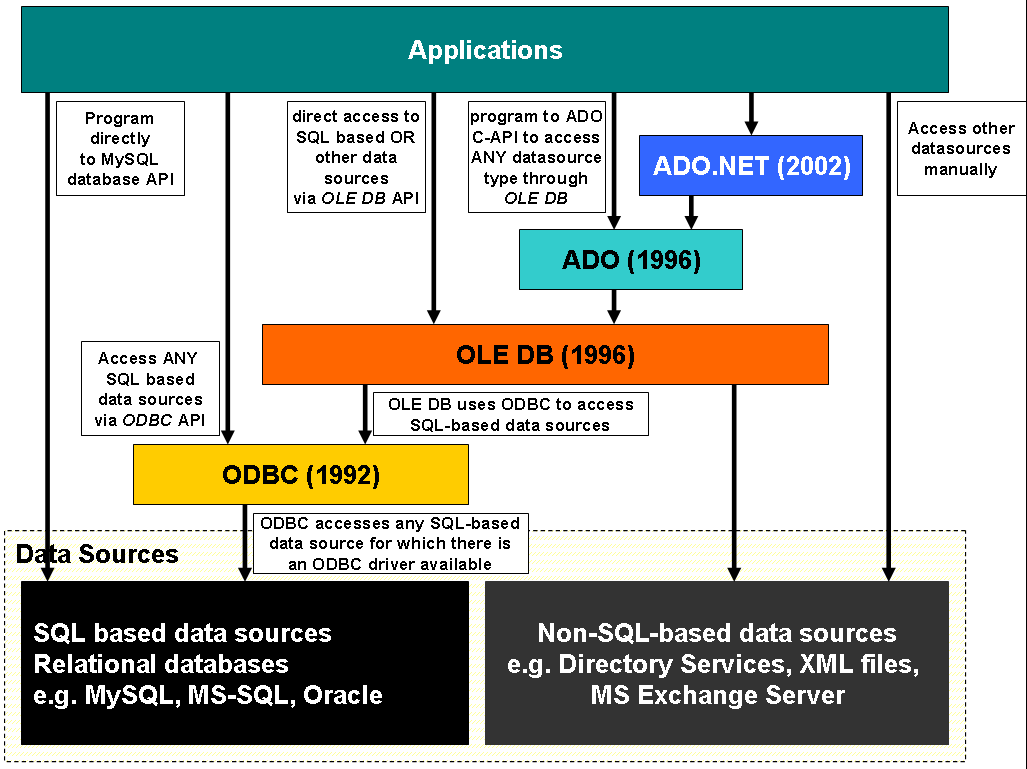
using System.Data.OleDB;
using System.IO;
static int Main(string[] args)
{
//Make a list to hold your params
List<string> paramList = new List<string>();
//Populate the list based on args
for (int i = 0; i < args.Length(); i++)
{
paramList.Add(args[i]);
}
//Create a new oledb connection
string path = "c:\\yourfilepath\\file.accdb";
OleDbConnection myConnection =
new SqlConnection("Provider=Microsoft.ACE.OLEDB.12.0;Data Source='" +
path + "';");
myConnection.Open();
//Run the stored proc on each param and output result to file
using (System.IO.StreamWriter file = new System.IO.StreamWriter(@"filepath\filename"))
{
foreach (string param in paramList)
{
//myCommand.CommandText is the query you want to run against your database
//i.e. the stored proc
OleDbCommand myCommand = myConnection.CreateCommand();
myCommand.CommandText = "stored_proc " + param;
OleDbDataReader myReader = myCommand.ExecuteReader();
if (myReader.HasRows)
{
file.WriteLine("pass: " + param);
}
else
{
file.WriteLine("fail: " + param);
}
myReader.Close();
}
}
myConnection.Close();
}