You're going to have to understand what a normal is in order to calculate the reflection vector on collison. Fortunately, it's not very hard to understand. For the math heads out there, I'm going to be a little imprecise, so please don't beat me up over it. :)
A normal is simply a unit vector (unit: having magnitude = 1) that's orthogonal (at a 90-degree angle) to a surface. You can visualize a normal as an arrow sticking straight out of a surface. Since your walls aren't slanted, figuring out the normals for them is easy (assuming the top-left corner of your screen is 0,0 here):
top: (0,1)
bottom: (0,-1)
left: (1,0)
right: (-1,0)
What we need to do is take the velocity of your ball and "reflect" it along the normal vector for the wall that you hit. The formula for reflection is the following:
V2 = V1 - 2*N*(N.dot(V1))
Where V1 is your incident vector (your old velocity in this case), N is the normal for the wall that we hit, and V2 is your reflected vector (your new velocity). "N.dot(V1)" is the "dot product" of N and V1, which is just (N.x*V1.x + N.y*V1.y).
A picture from Wikipedia of what we're doing (P is the incident vector, Q is the reflected vector):
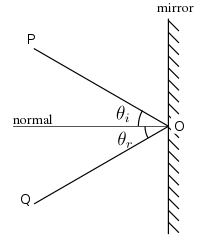
Here's the whole thing in psuedocode:
float nx = 0, ny = 1; // replace these with the normal of the wall you hit
float ix = ball.vx, iy = ball.vy; // incident vector
float dotprod = (nx*ix + ny*iy); // the dot product i mentioned earlier
ball.vx = ix - 2*nx*(dotprod);
ball.vy = iy - 2*ny*(dotprod);
Let me know if anything's unclear. :) Also, while this is kind of overkill for the walls, you will need to compute the normal when you do ball-ball collisions, so it's not a total waste...