There's no automated way I know of to have your plotted points change color based on the color of the pixel behind them. Keep in mind that you don't have to use just the eight predefined color specifications (i.e. 'r' for red or 'b' for blue). You could pick an RGB color specification for your plotted points that isn't common in your underlying image. For example:
h = plot(0,0,'Marker','x','Color',[1 0.4 0.6]); %# Plot a pink x
You could programmatically find the least common color with some simple code that picks the least frequently used color values in an image. Here's one example:
rawData = imread('peppers.png'); %# Read a sample RGB image
imData = reshape(rawData,[],3); %# Reshape the image data
N = hist(double(imData),0:255); %# Create a histogram for the RGB values
[minValue,minIndex] = min(N); %# Find the least used RGB values
plotColor = (minIndex-1)./255; %# The color for the plotted points
image(rawData); %# Plot the image
hold on;
hp = plot(500.*rand(1,20),350.*rand(1,20),... %# Plot some random points
'Marker','o','LineStyle','none',...
'MarkerFaceColor',plotColor,'MarkerEdgeColor',plotColor);
The above code first reshapes the image data into an M-by-3 matrix, where M is the number of image pixels and the three columns contain the red, green, and blue values, respectively. A binning of values is done for each column using HIST, then the value with the smallest bin (i.e. lowest frequency) is found for each column. These three values become the RGB triple for the plot color. When the image is overlaid with random points of this color, it gives the following plot:
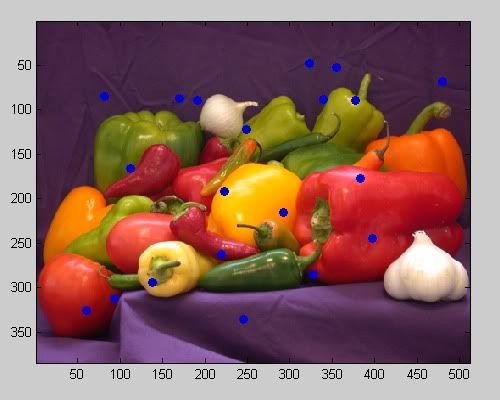
Notice in this case that the above code picks a bright blue color for the plot points, which happens to be a color not appearing in the image and thus giving good contrast.